How to Deploy a React App to a Subdirectory
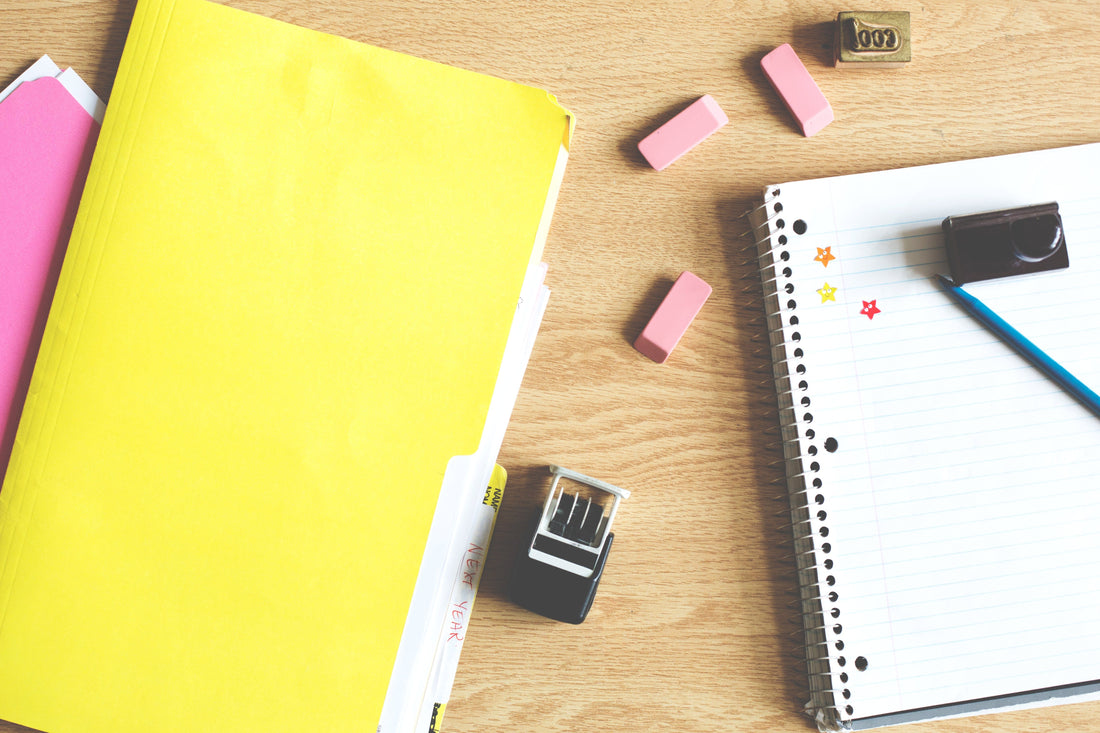
For the past month or so I've been working on a prototype app built with the React JavaScript library. It's been a lot of fun and I always enjoy the challenge of learning something completely new!
I got the app to a point where I wanted to show my teammates my progress in order to get some feedback on the usability and accessibility of the app. In order to do so, I had to deploy the production build of the app to a subdirectory on our web server.
After the initial deploy, I loaded up the app in my browser only to be met with a completely blank screen! What was happening here? I quickly jumped over to Google to help me figure this out.
Note: This post assumes you're using
create-react-app
andreact-router
v4+.
After a bit of searching around and reading some docs I was able to get things up and running! Here's what I found which will hopefully help you, dear reader, get your own React app up and running in a subdirectory! 🚀
1. Set the basename
Setting the basename
attribute on the <Router />
component tells React Router that the app will be served from a subdirectory.
<Router basename={'/directory-name'}>
<Route path='/' component={Home} />
{/* … */}
</Router>
Read more about using the basename
attribute over at ReactTraining.com.
2. Set the app homepage
Located in your package.json
file is a property called homepage
. The npm run build
command that comes with create``-``react-``app
uses this property in order to make sure the production build points to the correct location.
"homepage": "https://myapp.com/directory-name",
Read more on using the homepage
property from Create React App.
3. Update the Routes
You can also use the value set in the homepage
property discussed above in your JSX. This value is exposed in the process.env.PUBLIC_URL
variable at build time.
For example, I had to update the routes in my app to use the subdirectory on production by making the following changes:
<Router basename={'/subdirectory'}>
<Route path={`${process.env.PUBLIC_URL}/`} component={Home} />
<Route path={`${process.env.PUBLIC_URL}/news`} component={News} />
<Route path={`${process.env.PUBLIC_URL}/about`} component={About} />
</Router>
Note: this doesn't effect local development so it's safe to add to your project right away!
After making the changes above I was met with the home screen after a fresh build + deploy. Progress! Though, I noticed my <Link/>
s were still pointing to the domain root…
4. Update the Links
Scattered throughout the app were some <Link/>
components which I used to link from "page" to "page." These also needed the same treatment as the <Route/>
components:
<Link to={`${process.env.PUBLIC_URL}/page-path`}>…</Link>
With all these changes set in my app I was able to run npm run build
once more and deploy the changes to the server. At this point my app was up and running 💯 with no issues! 😀
I hope these notes help you to get your React app up and running in a subdirectory!
Did I miss anything you know of that would help make the process easier? Have any tips on setting the subdirectory path without making all these extra updates throughout? Please let me know as I'm still learning React! 🙌
Happy hacking! 💻😄💖