Getting Started with Webpack and ES6 Modules
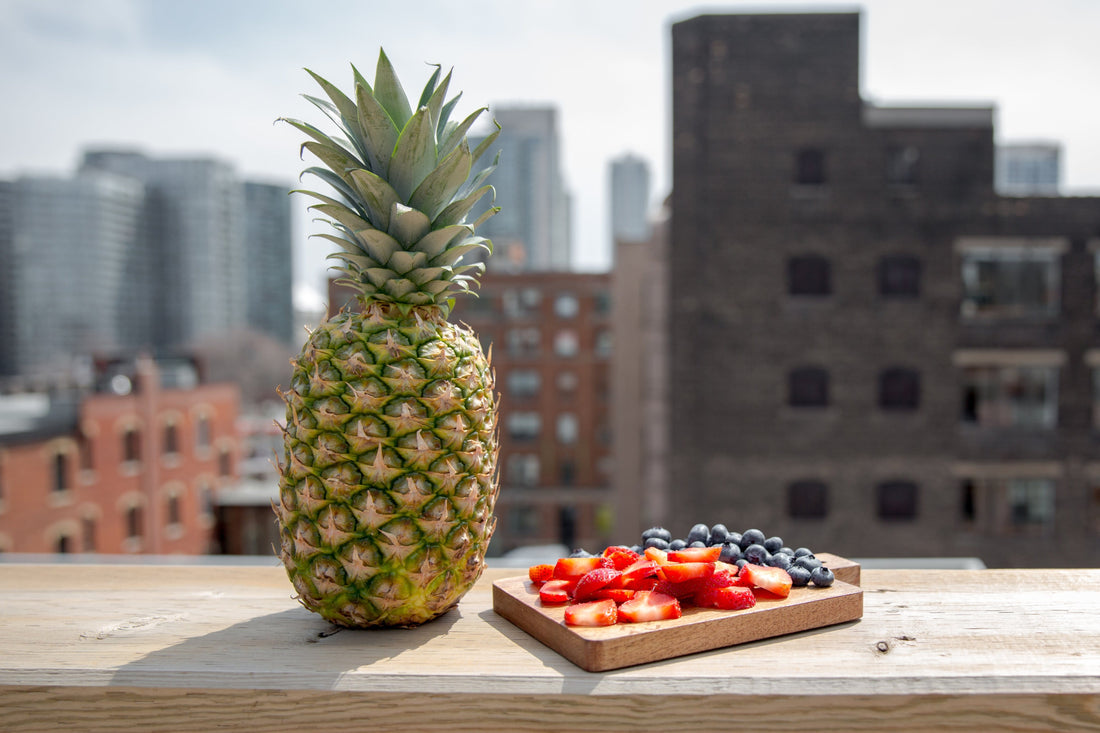
I used to think that webpack was one of those impossible to get started with technologies. Turns out, getting started with webpack and using ES6 modules isn't so bad!
Let's go through a tiny, very basic webpack based project. We'll look at the project structure, the configuration files, and a simple, high-level example of using webpack with ES6 modules.
- You can follow along with the demo project source code on GitHub !
- See the demo project in action !
Ready? Let's go! 🚀
Project structure
I'm a big fan of keeping things neat and organized, not just in my web projects but in real-life, too. Let's see how this demo project is setup:
- index.html
- package.json
- webpack.config.js
- /src
|- app.js
|- /modules
|- (various module files)
- /dist
|- bundle.js
-
index.html
is our HTML file which outputs the JavaScript content to the browser window. -
package.json
is our overall project configuration and meta data. This also has information on which third-party Node.js modules our project requires to work. More on this later. -
webpack.config.js
is where we tell webpack what to do, which files to read, and where to place the single JavaScript file that it creates. -
/src/app.js
is our working Javascript file. The is the base file where our changes will go, but we can also split our work into smaller files (modules)! -
/src/modules
holds the small, bit-sized, reusable pieces of our project. -
/dist
directory holds the output file, after webpack works its magic in bundling up all our smaller JavaScript files. -
/dist/bundle.js
is the file that webpack generates. It's the file that will be included in theindex.html
file.
Installing webpack
In order to make webpack available to use in the project, we just need to install it via npm
. Open up your Terminal app, change to your project directory, and type:
npm install webpack --save
webpack will be downloaded and added to your package.json
file { package.json:11 } ready to go.
Next let's look at the webpack.config.js
file.
webpack config
I'd say the most interesting part to pay attention to in the webpack config file is where we tell webpack our initial source file is, and where to place the output file when it bundles our Java Script files together.
Entry
The entry:
key { webpack.config.js:2 } lets webpack know, "Hey, this is the primary source file. Start here!" In the demo project, we tell webpack to use the app.js
file as the starting point.
entry: './src/app.js',
Output
The output:
key { webpack.config.js:3 } is used to specify the path and filename that we'd like webpack to generate. The bundle.js
file is the actual JavaScript that our project index.html
document { index.html:41 } will include and execute at run-time.
output: {
filename: './dist/bundle.js'
},
That's pretty much all we're concerned with when it comes to the webpack.config.js
file for our tiny demo project! There's more in this file you can explore but for now, let's move on and see how to use ES6 modules next.
Using ES6 modules
With webpack installed, setup, and ready to bundle our source code, we can now start using the import
and export
JavaScript keywords. These statements accomplish exactly what they sound like they would, "import JavaScript into this file so I can work with its code," and "export this JavaScript so it can be used elsewhere in the project."
Using the
import
andexport
statements is a way to split large files into smaller, reusable modules of code. It also helps to keep things neat and organized in the file structure!
Using export
For example, in the project structure there is a /src/modules/math-functions.js
file. This file contains a function called sum
{ math-functions.js:1 }.
const sum = (a, b) => {
return a + b;
};
The sum
function takes two numbers, adds them together, and returns the result. At the bottom of this same file, we see export {sum, ...};
{ math-functions.js:9 }.
export {sum, product};
This allows us to reuse the sum
function elsewhere in the project, but in order to do so we need to import
the function first.
Using import
Back at the top of the app.js
file, there area few import
statements { app.js:2 }.
import {sayHello} from './modules/greeting';
import {sum, product} from './modules/math-functions';
These allow us to use the functions, objects, or variables contained within our module files that we've exported.
The line which reads import {sum, ...} from './modues/math-functions';
will allow us to call the sum
function within the app.js
file as if it were defined within the same file! { app.js:12 }
const a = 3;
const b = 7;
// ...
resultSum.textContent = `The sum of ${a} and ${b} is ${sum(a, b)}. ✨`;
Pretty slick if you ask me. 😎
Putting it all together
The last piece of the puzzle is actually running the webpack
command in order to initiate the build and have webpack create the bundle.js
file.
We do this by setting up a script
entry in the package.json
file { package.json:6 }.
"scripts": {
"start": "npm run webpack",
"webpack": "webpack -d --watch"
},
Our custom command will run webpack in debug mode, which helps in providing more detail if something doesn't work right away in our project. This command also sets webpack to watch for any changes in the JavaScript files; when we make a change, webpack will re-bundle the bundle.js
file automatically for us!
So, right before you start working with JavaScript in the project, open your Terminal app, change to the project directory, and type npm start
. Watch as webpack reads the config and creates the bundle.js
file!
From here you can start making edits to the JavaScript files and load up index.html
in your browser to test your ES6 module code! 🎉
Was this guide helpful? Would you suggest anything else that I may have missed? Please let me know!
- Check out the demo project source code on GitHub !
- See the demo project in action !
Happy hacking! 💻😄💖